In the previous article, we discussed about basics of Node.js and its important terminologies. If you have not gone through the basics, I would recommend to look into it now and then proceed with this article.
In this article, we are going to create very simple app in node.js. In this application, we are going to create a simple request and response server application.
So, if you are little bit familiar with Node.js then you must have guessed it that we are talking about HTTP Server here. Node.js has in-built HTTP Module to server data and respond to client’s request.
But…..
First we need to create/initialize the project. So, choose your Pokemon(oops.. location) wisely and execute below command.
npm init
After running the above command, you will see a prompt to create a package.json file for your application. Fill up all the information but just pur entry point as app.js.
So, Let’s start writing code…
- Create a new file names “app.js” in root directory of project folder. This is the core file of the application which server pages and data.
Now, run the below command in Terminal
node app.js
Executing the above command and you will see below output in console
the server is running on http://localhost:3020
Go to your browser and type http://localhost:3020in the URL and v’olla our server is up and running. You might see something like below
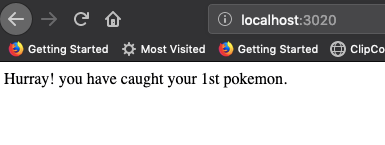
Running Some HTML Code
Now, we have just created one simple web server request and response, but it’s not that much. We want something more funny, some thing more challenging like printing some HTML response or printing some random number. For start, let’s try to print some HTML response. For this, I’m going to modify my “app.js” file with below code.
Let’s move one step further and learn how to send an HTML response with the web server. Modify the previous code to send the HTML response.
Before that, for sake of our time, Let’s first install nodemon npm package. This restart your Node.js Apps Automatically.
Execute below in your terminal
npm install -g nodemon
And, then run your app using nodemon instead of node. like below:
nodemon app.js
Now, you have to just save your code and refresh your browser. All of your code will be loaded automatically.
Now, we also need to add the additional setting that indicates the MIME type of the response. There is so many MIME type which a server can send to the client. For demo purpose, let’s use “text/html” MIME type. So After modifying the code now, Our code looks like below:
In the above code, we added some HTML tag and MIME type with the response.
Now refresh your browser, you will see HTML response in the browser like below image
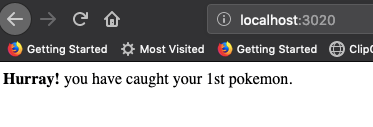
So, we learn how to send plain and HTML response using Node.js server but as a developer or in any web application development we do not want to write code in such way. For our next series, we will learn some frameworks to build our web applications.